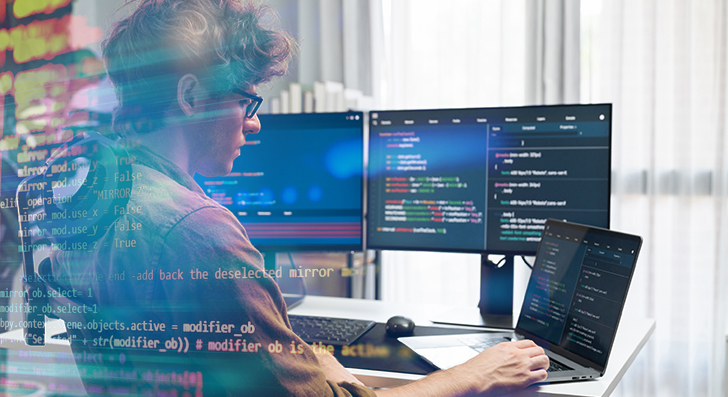
Scalability usually means your application can deal with growth—extra end users, a lot more data, and more traffic—without breaking. For a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and realistic guidebook that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be part of your respective strategy from the start. Numerous apps fail if they develop rapid simply because the first design and style can’t tackle the extra load. Being a developer, you have to Consider early regarding how your program will behave stressed.
Begin by coming up with your architecture to be versatile. Stay clear of monolithic codebases exactly where anything is tightly connected. As an alternative, use modular structure or microservices. These patterns break your application into smaller, impartial parts. Just about every module or service can scale on its own devoid of affecting The entire technique.
Also, think about your database from working day one. Will it want to manage one million users or perhaps a hundred? Select the ideal type—relational or NoSQL—according to how your info will improve. Plan for sharding, indexing, and backups early, even if you don’t require them but.
One more significant issue is to avoid hardcoding assumptions. Don’t generate code that only works under current circumstances. Take into consideration what would take place When your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design designs that guidance scaling, like concept queues or celebration-pushed programs. These support your app manage far more requests devoid of finding overloaded.
Any time you Make with scalability in your mind, you're not just preparing for success—you might be lessening long run complications. A properly-planned method is easier to take care of, adapt, and improve. It’s superior to organize early than to rebuild later.
Use the Right Databases
Picking out the correct database is usually a essential Portion of creating scalable applications. Not all databases are crafted the exact same, and using the Incorrect you can sluggish you down and even cause failures as your application grows.
Start off by comprehending your data. Could it be extremely structured, like rows inside of a table? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically robust with interactions, transactions, and consistency. In addition they assist scaling methods like examine replicas, indexing, and partitioning to deal with additional site visitors and details.
When your data is much more adaptable—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing huge volumes of unstructured or semi-structured data and may scale horizontally extra very easily.
Also, take into consideration your go through and produce patterns. Have you been accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you currently dealing with a major create load? Look into databases that will cope with high compose throughput, or maybe event-primarily based knowledge storage units like Apache Kafka (for temporary info streams).
It’s also sensible to Imagine in advance. You may not want Innovative scaling options now, but choosing a database that supports them implies you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your data based on your accessibility patterns. And constantly watch databases general performance when you mature.
To put it briefly, the right databases relies on your application’s composition, velocity desires, And just how you be expecting it to increase. Just take time to choose properly—it’ll conserve lots of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, just about every modest hold off adds up. Badly created code or unoptimized queries can slow down general performance and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Begin by writing clean up, basic code. Stay away from repeating logic and remove just about anything unwanted. Don’t select the most complicated Alternative if a straightforward just one performs. Keep your capabilities limited, focused, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code usually takes also lengthy to operate or makes use of too much memory.
Upcoming, take a look at your databases queries. These usually gradual items down more than the code by itself. Make sure Just about every query only asks for the information you truly need to have. Steer clear of Pick out *, which fetches every thing, and as a substitute choose precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, Primarily across substantial tables.
If you observe the identical details becoming asked for many times, use caching. Retailer the final results quickly utilizing instruments like Redis or Memcached so that you don’t should repeat expensive operations.
Also, batch your database functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and would make your application much more productive.
Make sure to exam with large datasets. Code and queries that perform wonderful with a hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when needed. These actions aid your application keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage additional buyers plus more traffic. If everything goes via 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching come in. Both of these applications enable maintain your app quickly, stable, and scalable.
Load balancing spreads incoming site visitors across numerous servers. As opposed to 1 server performing all of the work, the load balancer routes users to distinctive servers based upon availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing details quickly so it could be reused swiftly. When consumers request a similar facts yet again—like a product page or maybe a profile—you don’t ought to fetch it in the databases anytime. You'll be able to provide it with the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapid accessibility.
2. Customer-side caching (like browser caching or CDN caching) outlets static information near the consumer.
Caching cuts down database load, increases speed, and will make your app extra productive.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when information does improve.
In a nutshell, load balancing and caching are simple but strong tools. Collectively, they assist your app take care of extra consumers, keep fast, and Recuperate from problems. If you intend to mature, you'll need each.
Use Cloud and Container Equipment
To make scalable applications, you will need instruments that permit your app develop simply. That’s wherever cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and services as you'll need them. You don’t must acquire hardware or guess foreseeable future ability. When website traffic improves, you can add much more resources with just a few clicks or immediately utilizing automobile-scaling. When visitors drops, you'll be able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your application in lieu of managing infrastructure.
Containers are another vital Resource. A container deals your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from the laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to individual areas of your app into expert services. You'll be able to update or scale parts independently, and that is great for general performance and dependability.
To put it briefly, employing cloud and container tools suggests you are able to scale speedy, deploy very easily, and Get better swiftly when complications take place. If you prefer your app to mature with out boundaries, commence working with these resources early. They help save time, decrease possibility, and enable you to stay focused on setting up, not fixing.
Keep an eye on Everything
Should you don’t here watch your application, you won’t know when factors go wrong. Monitoring helps you see how your app is undertaking, spot concerns early, and make greater conclusions as your application grows. It’s a important Section of setting up scalable methods.
Commence by tracking primary metrics like CPU use, memory, disk House, and response time. These tell you how your servers and providers are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application too. Keep an eye on how long it will take for consumers to load webpages, how frequently glitches transpire, and where they happen. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Create alerts for crucial difficulties. By way of example, If the reaction time goes earlier mentioned a Restrict or maybe a assistance goes down, it is best to get notified promptly. This can help you deal with troubles rapidly, usually prior to customers even notice.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you can roll it again just before it leads to real problems.
As your app grows, traffic and details enhance. Without having checking, you’ll miss out on signs of hassle till it’s much too late. But with the best resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application dependable and scalable. It’s not almost spotting failures—it’s about being familiar with your program and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for huge companies. Even modest applications want a solid foundation. By coming up with cautiously, optimizing correctly, and using the appropriate applications, it is possible to Establish apps that improve smoothly with no breaking stressed. Commence smaller, think massive, and Establish wise.